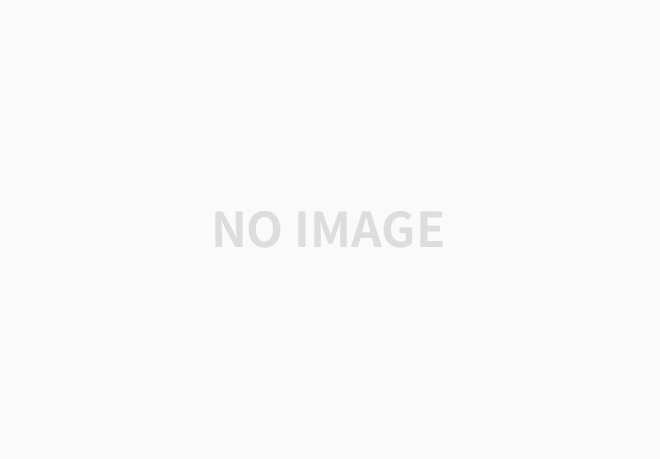
❗matches 함수의 기본적인 사용방법
- matches 함수는 Element의 내장 메서드입니다.
- CSS 선택 문자열로 요소의 일치 여부를 판단할 수 있습니다.
const element = document.getElementById('myElement');
if (element.matches('.myClass')) {
console.log('The element matches the selector');
} else {
console.log('The element does not match the selector');
}
❗matches를 이용한 버튼 이벤트 추가
- 다음과 같이 버튼이 있다고 했을 때, 이벤트를 어떻게 부여할 수 있을까요?
<div id="select">
<button data-test="info1">btn1</button>
<button data-test="info2">btn2</button>
<button data-test="info3">btn3</button>
</div>
- 기본적으로 순회를 통해서 addEventListener를 부여할 수 있지만, JS의 Element에 사용할 수 있는 함수 matches()를 사용할 수도 있습니다.
- matches 메서드는 매개변수로 Selector 문자열을 받습니다.
const TEST_BTN_SELECTOR = 'button[data-test]';
document.getElementById("select").addEventListener('click', (e) => {
const {target} = e;
if(target.matches(TEST_BTN_SELECTOR)){
console.log(target.dataset) // 클릭한 버튼의 data-test의 value
}
})
- dataset을 이용한 Selector 문자열은 “요소태그[dataset]” 으로 만들 수 있습니다.
❗Select는 Change이기 때문에 matches 메서드를 사용할 수 없다.
- 버튼과 달리 chnage 이벤트를 발생하기 때문에 matches() 함수를 사용하여 이벤트를 추가할 수 없습니다. 대신 target의 value 값이 선택된 option의 innerText를 반환합니다.
<body>
<select id="select">
<option>btn1</option>
<option>btn2</option>
<option>btn3</option>
</select>
</body>
document.getElementById("select").addEventListener('change', (e) => {
const {target} = e;
console.log(target.value)
})
반응형
'개발관련 > Javascript' 카테고리의 다른 글
가비지 컬렉션 정리 (0) | 2024.11.21 |
---|---|
[JS] 상속받은 서브 클래스에서의 this에러 (0) | 2024.02.14 |
[Javascript] Object.freeze()된 객체는 변경할 수 없을까? (0) | 2023.11.10 |
[Javascript] If 조건문과 Switch 조건문의 차이 (0) | 2023.11.07 |
[Javascript] Jest 실패한 테스트만 실행시켜보자. watch 모드 기능 (0) | 2023.11.03 |