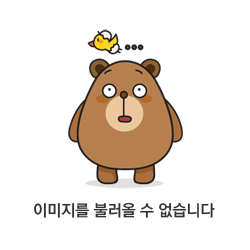
https://www.acmicpc.net/problem/2573
- Bfs함수를 따로 만들고, 시간이 한번 지날때 바뀌는 빙산의 모양을 만드는 nextYear함수를 따로 만들어서 공략했습니다.
- 함수를 따로 만들면 이해하기가 쉽습니다.
let fs = require('fs');
let input = fs.readFileSync('/dev/stdin').toString().trim().split("\\n")
const [N,M] = input.shift().split(" ").map(Number)
let iceberg = input.map(v => v.split(" ").map(Number))
const next = [[1,0],[-1,0],[0,1],[0,-1]]
let count = 0
while(true){
const answer = findBfs()
if(answer >1){
break;
}else if(answer === 1){
nextYear()
count++
}else if(answer === 0){
count = 0
break;
}
}
console.log(count)
//1년이 지남에 따른 빙산 변화를 나타내는 함수
function nextYear () {
const newIceberg = JSON.parse(JSON.stringify(iceberg))
for(let i=1; i<N-1; i++){
for(let j=1; j<M-1; j++){
if(iceberg !== 0){
const old = iceberg[i][j]
let count = 0;
for(let [a,b] of next){
if(iceberg[i+a][j+b] === 0){
count++
}
}
newIceberg[i][j] = old-count > 0 ? old-count : 0
}
}
}
iceberg = newIceberg
}
//BFS를 이용하여 빙산의 개수를 구하는 함수
function findBfs () {
const bfs = Array.from({length:N},()=>Array(M).fill(-1))
let count = 1;
for(let i=1; i<N-1; i++){
for(let j=1; j<M-1; j++){
if(bfs[i][j] === -1 && iceberg[i][j] !== 0){
bfs[i][j] = count
const Queue = [[i,j]]
let order = 0;
while(Queue.length > order){
const [a,b] = Queue[order]
for(let [a2,b2] of next){
if(iceberg[a+a2][b+b2] !== 0 && bfs[a+a2][b+b2] === -1){
Queue.push([a+a2,b+b2])
bfs[a+a2][b+b2] = count
}
}
order++
}
count++
}
}
}
return count-1
}
반응형
'개발관련 > 백준' 카테고리의 다른 글
백준 JS 2457번 공주님의 정원 그리디 (0) | 2023.06.23 |
---|---|
백준 JS 1600번 말이 되고픈 원숭이 BFS (0) | 2023.06.23 |
백준 JS 1005번 ACM Craft. DP (0) | 2023.06.09 |
백준 JS 2565번 전깃줄 DP (0) | 2023.06.09 |
백준 JS 1520번 내리막 길. DP + DFS (0) | 2023.06.09 |